The Raspberry Pi is a single-board computer that was created to make computing accessible to everyone. With a plethora of accessories and global community support, it serves as a gentle introduction to physical computing.
Python is one of the most popular programming languages in the world, and is an integral part of the Raspberry Pi. Let’s take a closer look at its data structures and commands.
Creating Comments in Code With #
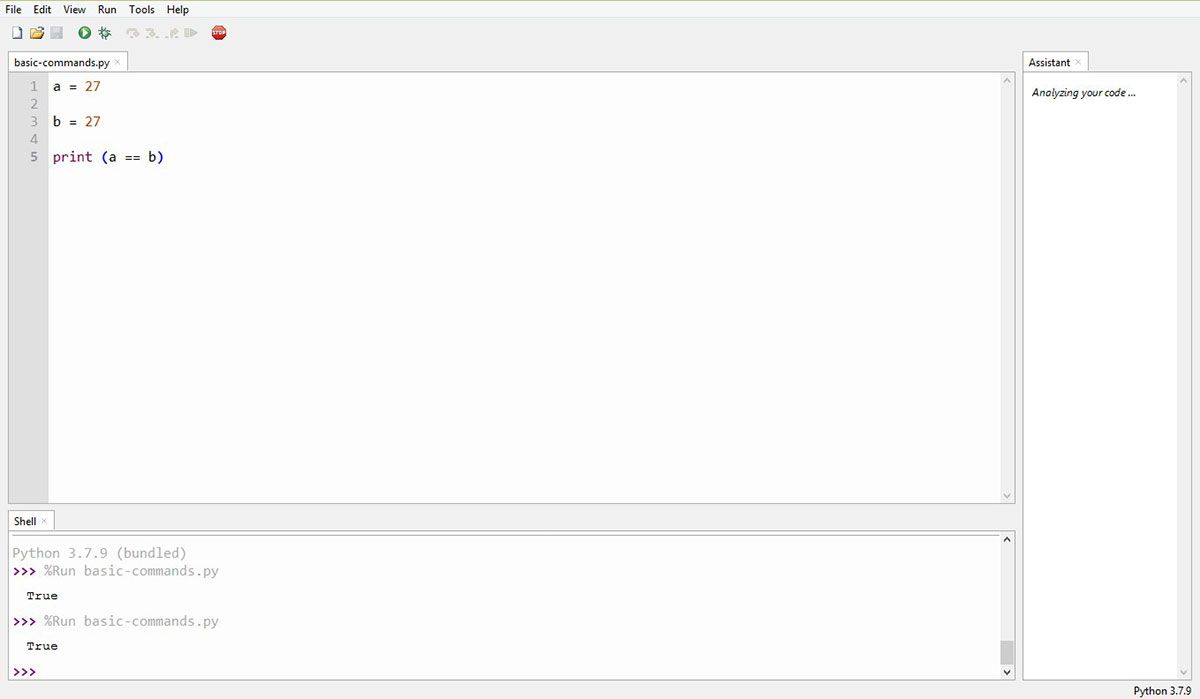
As a project’s scope increases, so can the complexity of the code. Making code easily readable is a priority, that's why there's colour coding in IDEs, and there are a number of great IDE choices for programming on the Raspberry Pi.
To make it even easier to read, programmers use comments in their code, which is text intended for other developers or humans to understand. In Python, a comment can be made by using a hash character, #, at the start of a comment. For example:
# This is a comment!
Import a Module in Python
The import keyword or command lets you access other modules in Python. There are many modules in Python which have powerful features. For instance, the math module lets you access mathematical functions to be used in your code:
import math
Using the Print Command With Data Types
Previously, we’ve seen examples of values used in Python code such as the string, “Hello World!”. These values can be categorised into data types.
Data Types: Numbers
A powerful feature of programming is the ability to manipulate variables. Variables can be thought of as containers that hold a value. In other words, a name that refers to a value. For example the integer data type is seen here, where a = 27. Here, the variable a is declared with an integer value of 27.
a = 27
The assignment operator assigns a value to a variable, a, with a value of integer data type.
Data Types: Strings
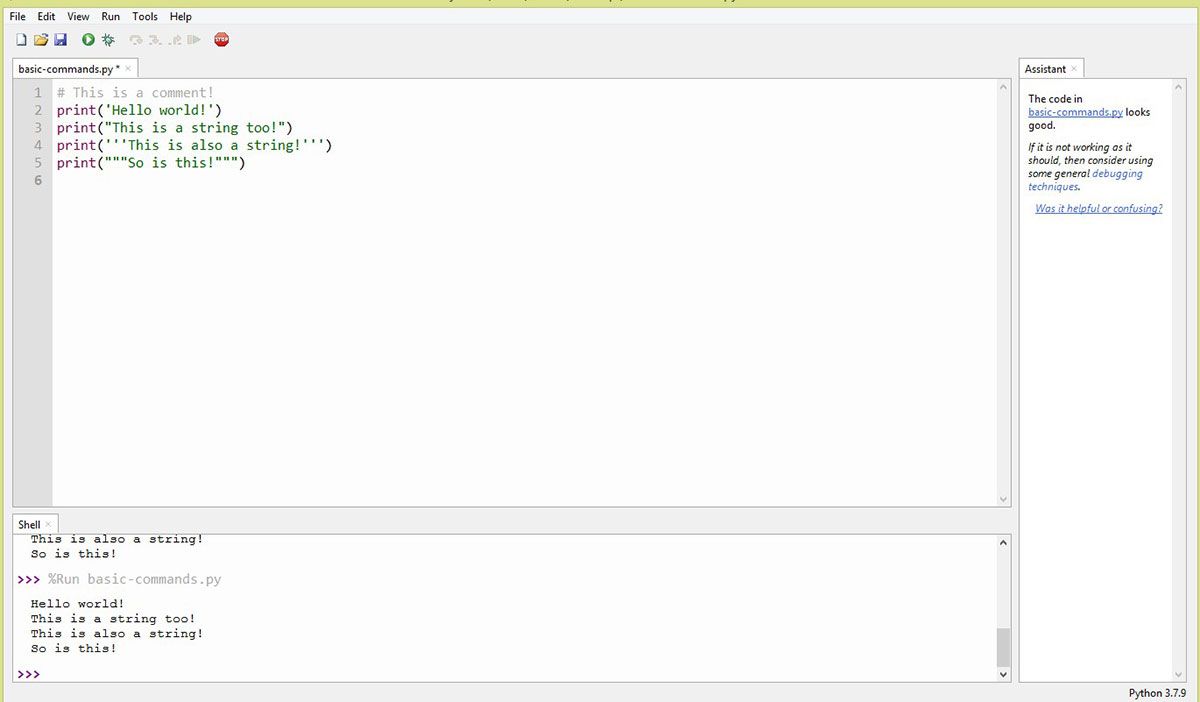
The string data type are Unicode characters enclosed within single, double, or triple quotes. The examples below are all strings that are printed to the console with the print command.
print(‘Hello World!’)
print(“This is a string too!”)
print(‘’’This is also a string!’’’)
print(“””So is this!”””)
That's not all you can do with strings! Besides printing them, there are plenty of other ways to manipulate strings in Python.
Data Types: Boolean
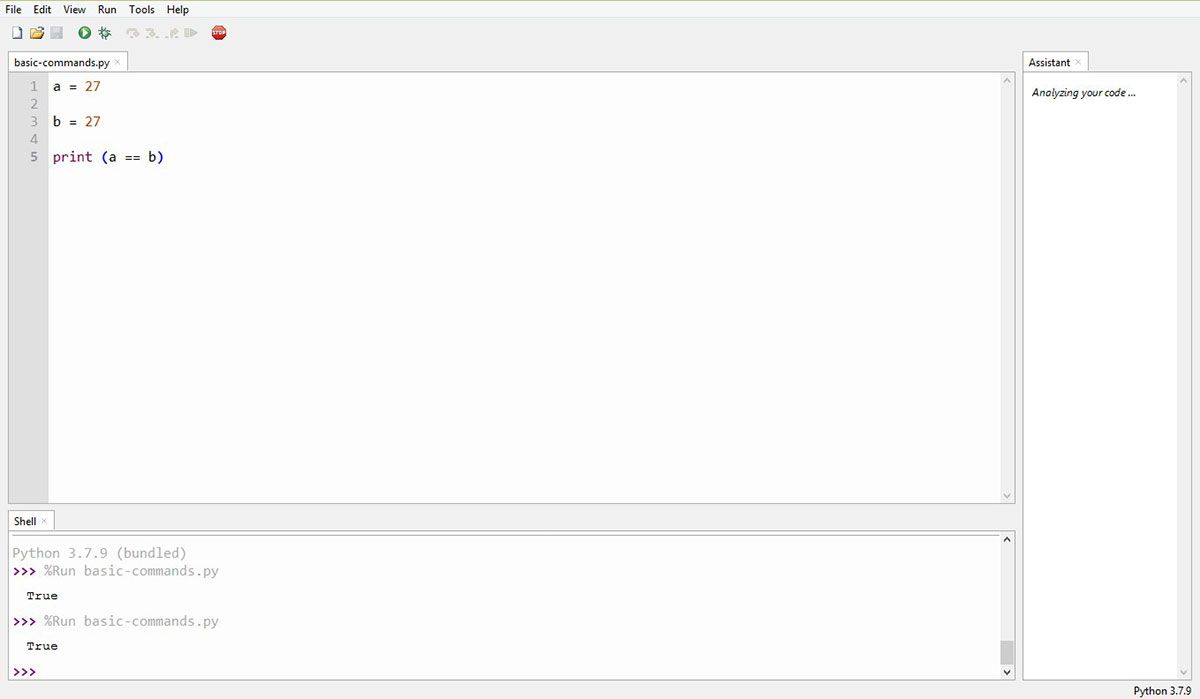
Another data type used in Python is the Boolean, used to represent the truth value of an expression. These values can be either true or false, let’s take a look at the following example:
a = 27
b = 27
print (a == b)
Here the variable a is compared to the variable b; Since they are both equal in value, it results in a value of True. Its usefulness can be further seen in the case of validating strings using Boolean methods. That is to say, you can use Boolean validation to manipulate strings in Python.
Data Types: List
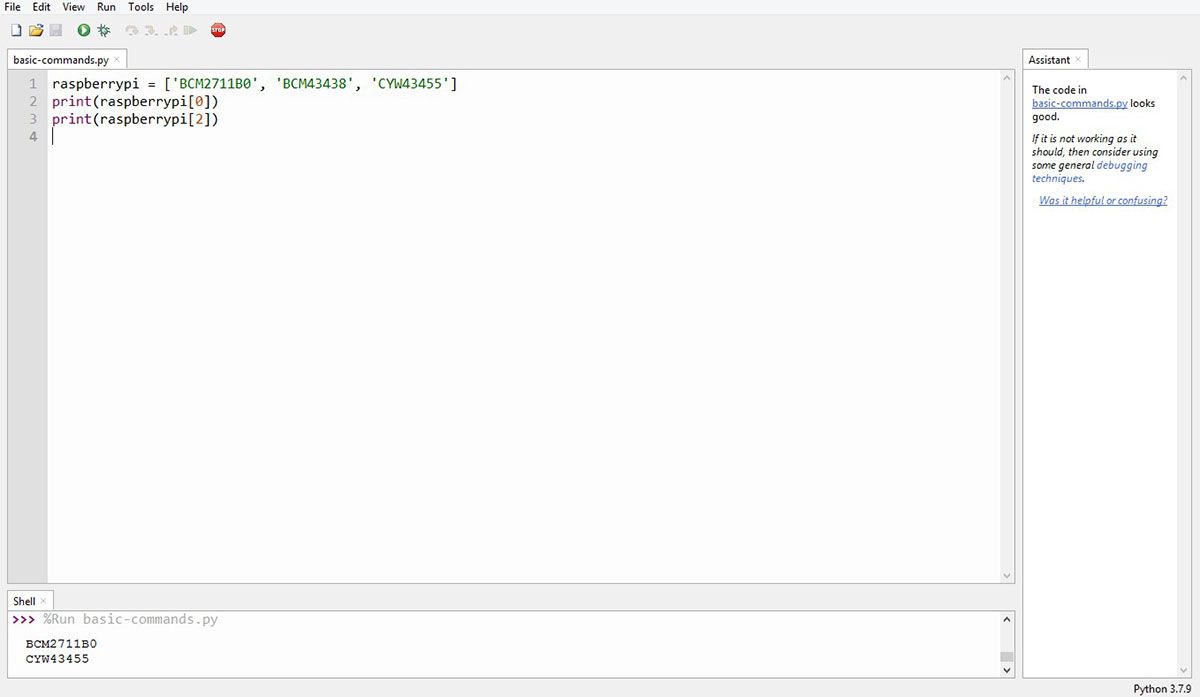
Lists are a collection of values rather than a single value and come in useful when you need to keep data for later computation. Defining a list in Python can be done by assigning a number of objects to a variable name using the = operator. For instance:
raspberrypi = [‘BCM2711B0’, ‘BCM43438’, ‘CYW43455’]
The list of values will need to be within the ‘[‘ and ‘]’
To print the value at (for example) index 0, use the command:
print(raspberrypi[0])
To print the value at index 2, use the command:
print(raspberrypi[2])
Data Types: Dictionary
Other times, it may be necessary to store collections of values and know just where they are placed. A Python dictionary can be used for this as it stores a key and value pair; it is also ordered and changeable. Use the braces (‘{‘ and ‘}’) notation to create a dictionary like so:
bom = {‘raspberrypi’ : ‘2’ , ‘capacitor’ : ‘20’ , ‘pushbuttons’ : ‘20’ , ‘LEDs’ : ‘20’}
To return and print an object that displays a list of all keys in the dictionary in order of insertion, use the keys() method like so:
print(bom.keys())
On the other hand, to retrieve and print all the values from a dictionary, use the values() method like so:
print(bom.values())
Data Types: Tuple
Similar to lists, tuples are collections of values. However, they are immutable which means that they are unchangeable. A tuple can be created by using parentheses:
MUO = (‘PC’ ,’Mobile’, ‘Lifestyle’ ,’Hardware’, ‘Free Stuff’, ‘Deals’)
Besides strings, a tuple can also store lists like so:
MUO = ([‘Technology Explained’, ‘Buyer’s Guides’, ‘Smart Home’ ,’DIY’, ‘Product Reviews’])
Conditional Logic: If-Else Statements
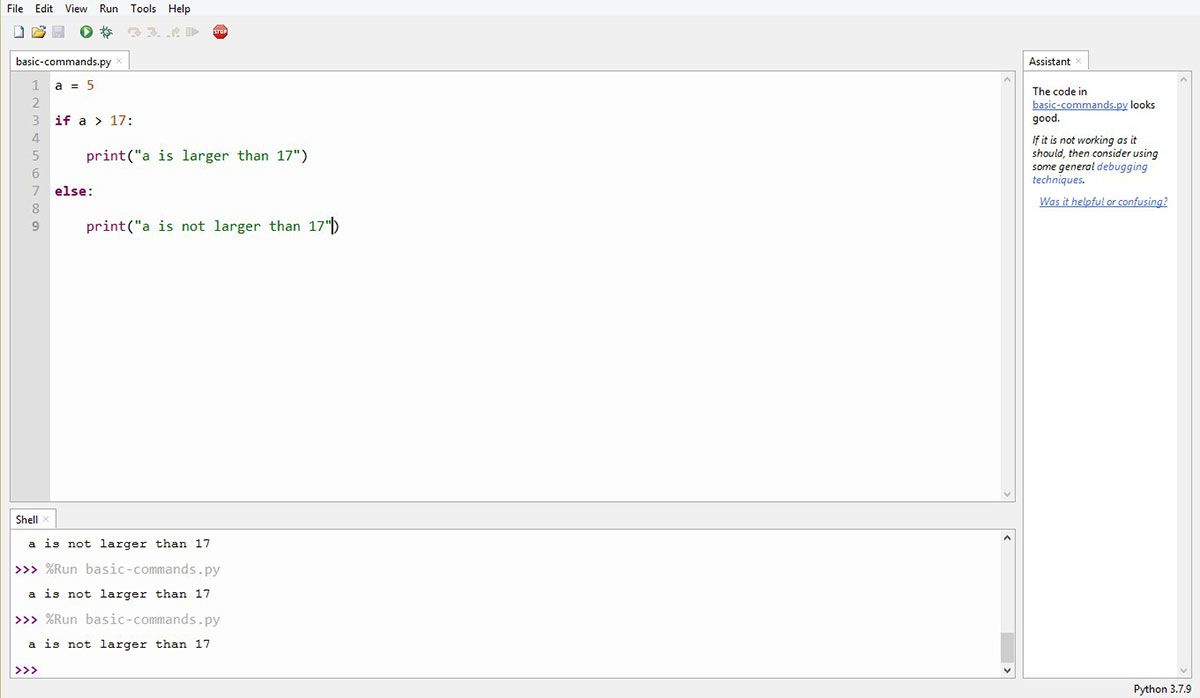
In order to write useful programs, conditional logic is required. One of the simplest forms can be found in the if statement. Before delving into conditional statements, it helps to take a closer look at indentation. Indentation are the leading whitespaces as shown in the example:
a = 5
if a > 17:
print(“a is larger than 17”)
In this case, the indented print statement executes if the statement returns true. Use four consecutive spaces for a level of indentation.
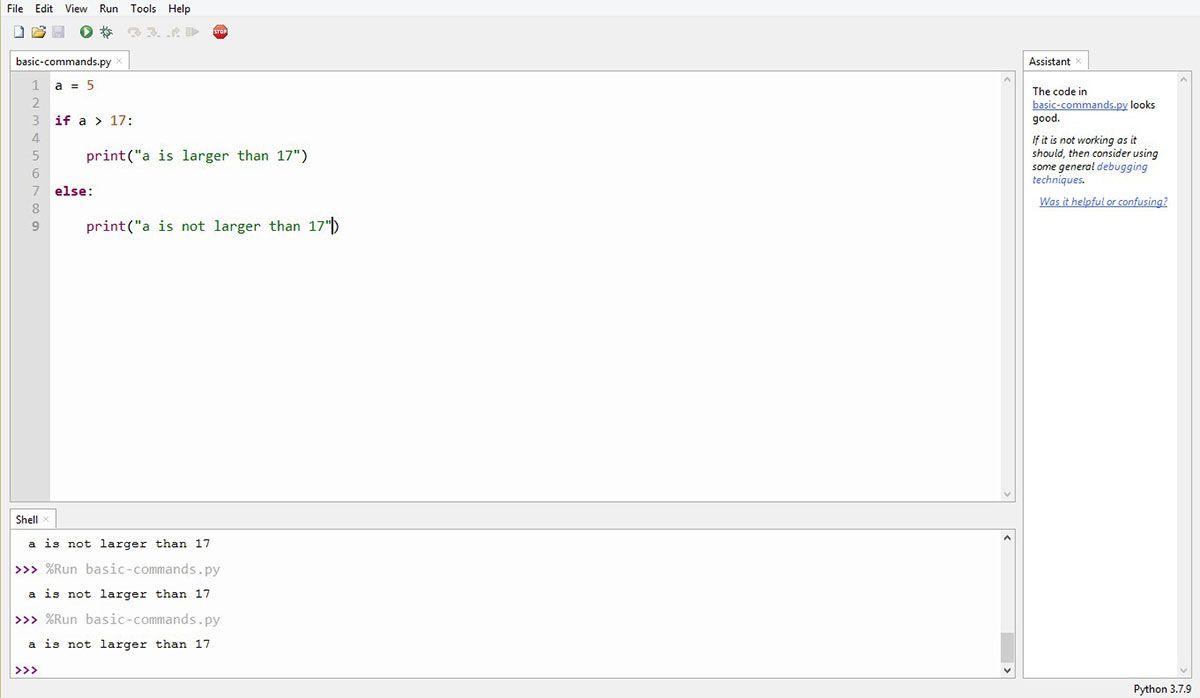
In this next example, the else statement is used to print “a is not larger than 17”. Since the first condition is not true, the statement under the else clause gets executed instead.
a = 5
if a > 17:
print(“a is larger than 17”)
else:
print(“a is not larger than 17”)
Loops: For Statements
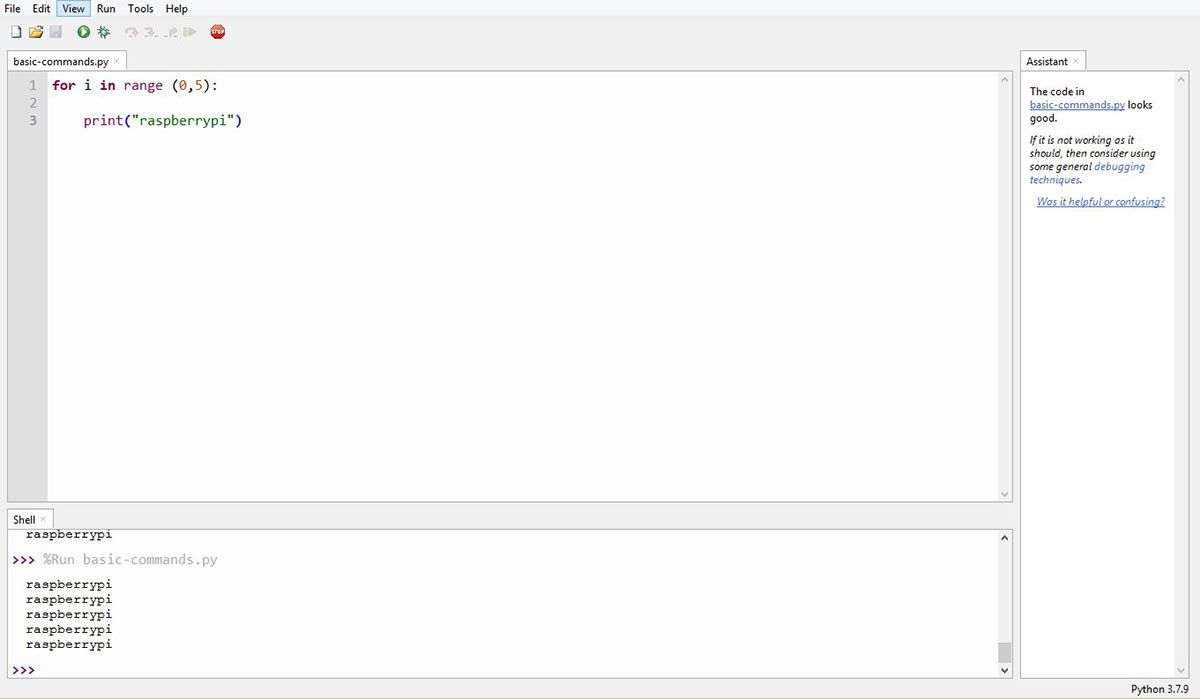
The for statement is used when there is a block of code that needs to be repeated a number of times. For example, here the word ‘raspberrypi’ is printed five times:
for i in range (0,5):
print(“raspberrypi”)
Loops: While Statements
To repeat a block of code over and over again, use the while statement. These are controlled by a conditional expression. In this example, the following will continue to be printed:
while (True):
print(“raspberrypi”)
Break Command
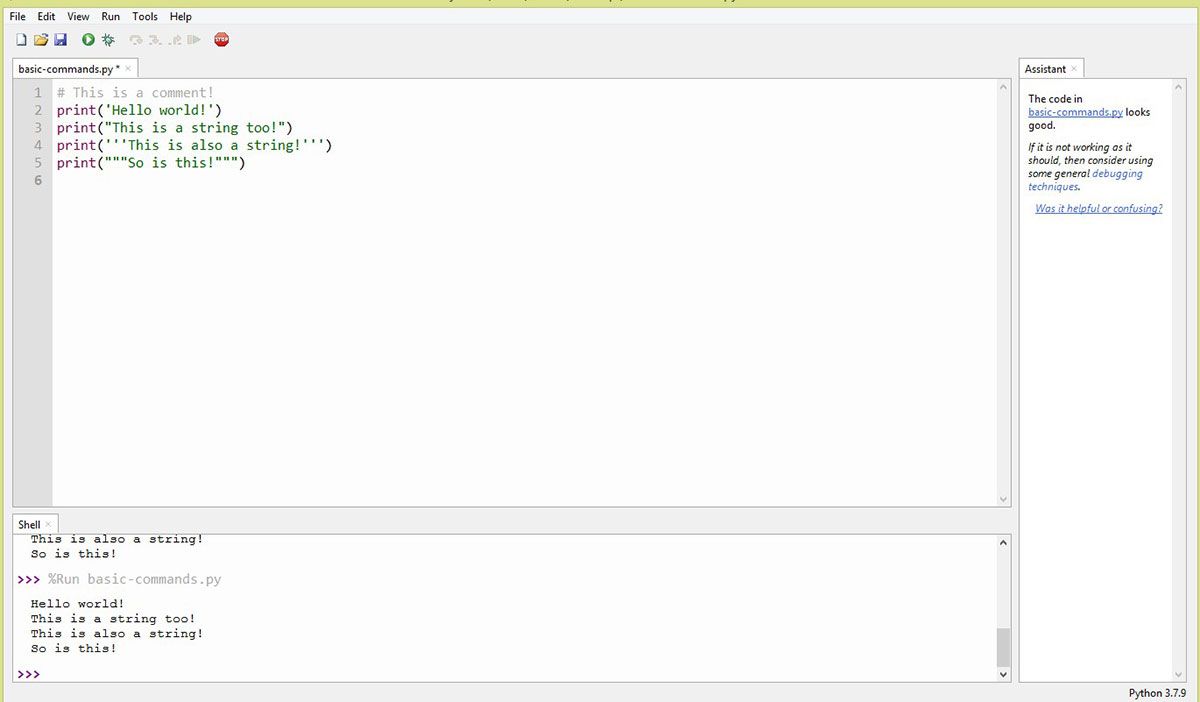
Sometimes you want a loop to stop executing, to do so, a break statement can be enclosed within an if statement. For example:
x = 0;
while (True):
print(“raspberrypi”)
x += 1
if x > 20:
break
Create More With Raspberry Pi
This article has briefly gone through some of the basic data structures and commands in Python. There are many more commands and modules, so you may want to have a read through the official Python documentation. Be sure to also check out the Python FAQs for support and troubleshooting.